The correct, complete, 2024, settings to configure the Java version in Gradle for Android.
Setting JDK level in Android Gradle builds
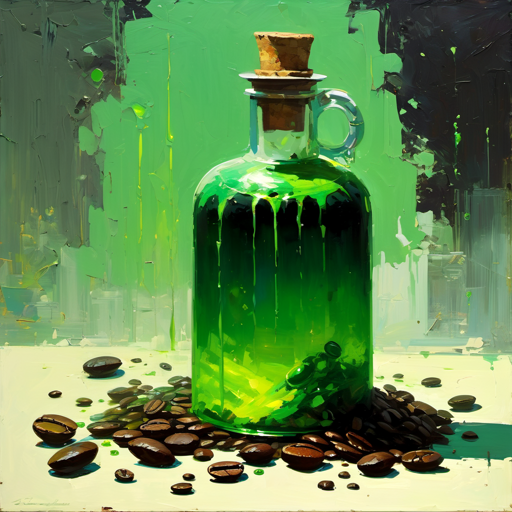
JDK version issues always confuse me. If you don’t set the right Java version level for your project, you come across a whole plethora of mysterious errors at the most inconvenient times. I switch project that infrequently that it’s hard to remember how it was fixed last time!
So here I am writing down how to set the Java level for your Android project. That is; how to set the JVM target, how to set your project Java compatability, how to set your target Java version, how to choose your Java toolchain, what version of the JVM your Android project uses, how to choose Java in Gradle Android … you get the idea, however you want to describe it, this is it!
All of this information is already out there and explained thoroughly, the problem is it’s changed repeatedly throughout the years and Google tends to enjoy indexing old things. Therefore I’m writing this as a personal TLDR.
As of Jan 2024 I’ve found I typically want to be setting your Java version to 17 if possible. If you are finding you have other compatibility issues when doing this, then go with Java 11 but spend some time writing down what isn’t working and tackling those issues as well, then bump to Java 17. If you are still targeting Java 8 … I wish you well in your struggles.
When setting the Java version for your Android Gradle project. Each module is the same, it’s no different for the Android application module than it is for any Android library modules. You need to set this declaration for every module independently. You could look into pulling this duplication out into a composite build or custom gradle plugin, but that’s another blog entirely.
The Code
For each module:
In the android block:
android {
...
compileOptions {
sourceCompatibility JavaVersion.VERSION_17
targetCompatibility JavaVersion.VERSION_17
}
kotlinOptions {
jvmTarget = "17"
}
...
}
And outside the android block on its own:
kotlin {
jvmToolchain(17)
}
References to the above Gradle options:
1) sourceCompatibility / targetCompatibility
2) jvmTarget
3) Android docs jvmToolchain, Gradle docs jvmToolchain
(Assuming you are running Gradle >= v6.7 & Kotlin >= v1.7.2.)
N.b. If you have any Java only modules, your gradle file only needs to include:
java {
toolchain {
languageVersion = JavaLanguageVersion.of(17)
}
}
N.b. If you have any Kotlin (& Java) only modules, your gradle file only needs to include:
kotlin {
jvmToolchain(17)
}
As well as avoiding errors, setting explicit Java versions for all modules will clear up warnings about Java targets being misaligned, such as:
'compileDebugJavaWithJavac' task (current target is 11) and 'kaptGenerateStubsDebugKotlin' task (current target is 1.8) jvm target compatibility should be set to the same Java version.
By default will become an error since Gradle 8.0+! Read more: https://kotl.in/gradle/jvm/target-validation
Consider using JVM toolchain: https://kotl.in/gradle/jvm/toolchain
And that’s it, as long as you do that for every module in your project you should have a consistent Java version.
Any questions, you can find me on:
Threads @Blundell_apps